Desert Bloom, conceived as a forward-looking resource harvesting concept, addresses the escalating impact of climate change and extreme environmental conditions. This system harnesses solar power, collects rainwater, and, equipped with distance sensors, autonomously safeguards its resources. Inspired by nature, the design mimics a flower – opening its petals to capture sunlight and repositioning them during rain to channel water into an internal reservoir.
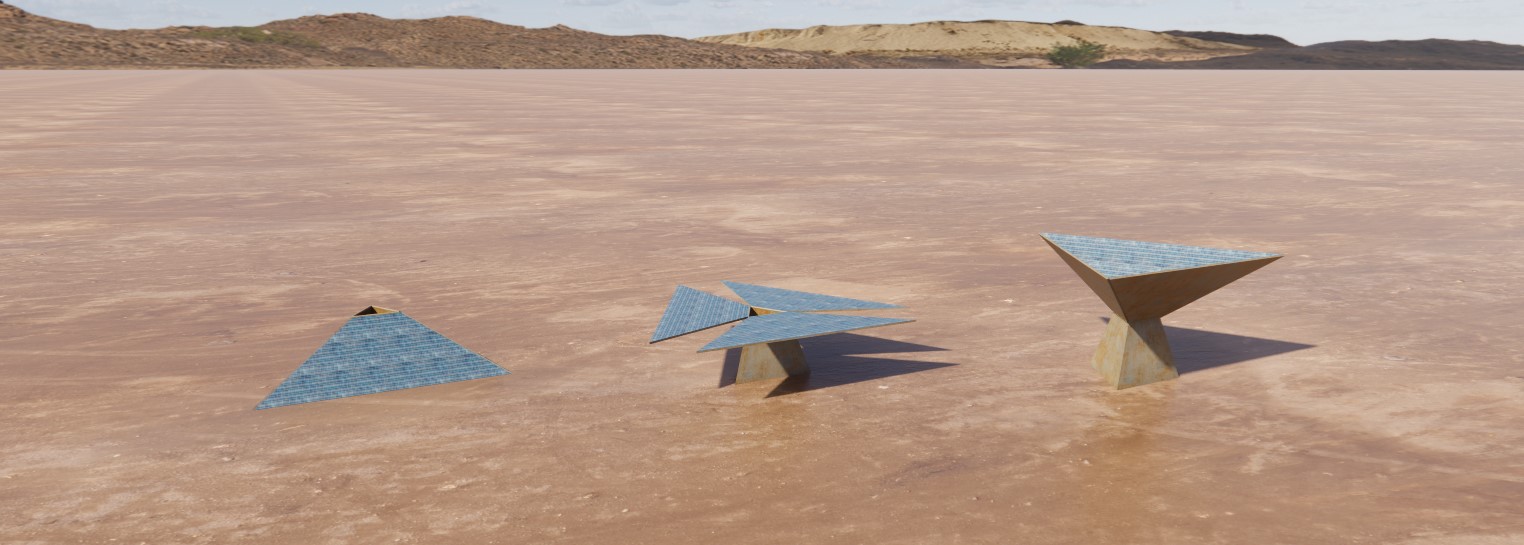
Bill of Materials/Circuit Diagram
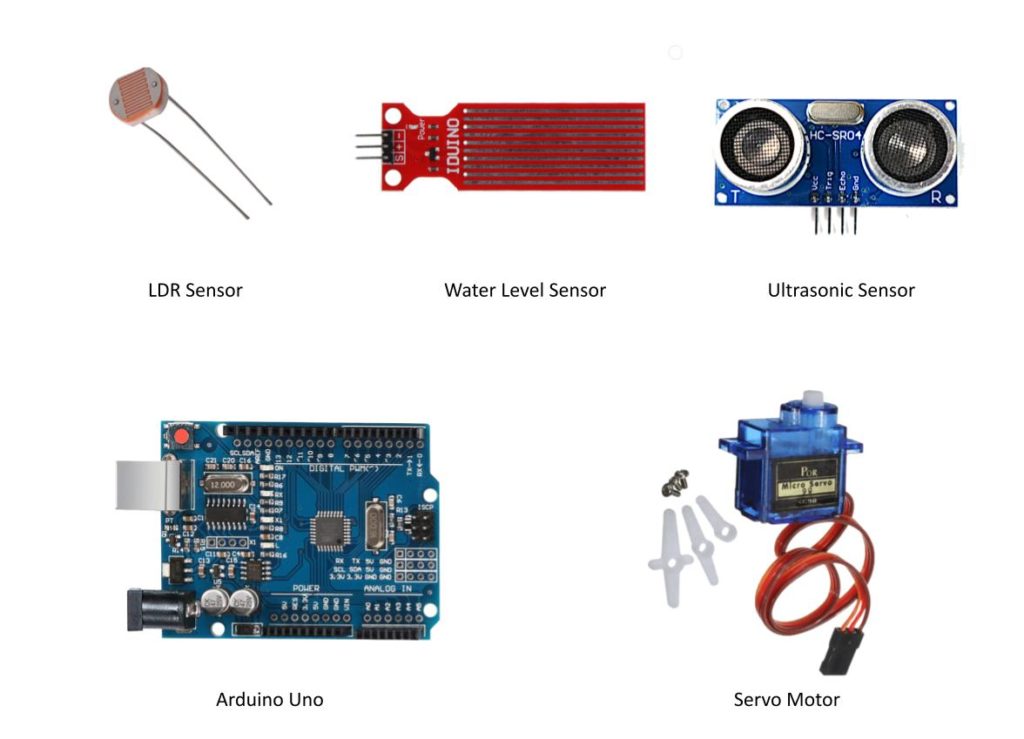
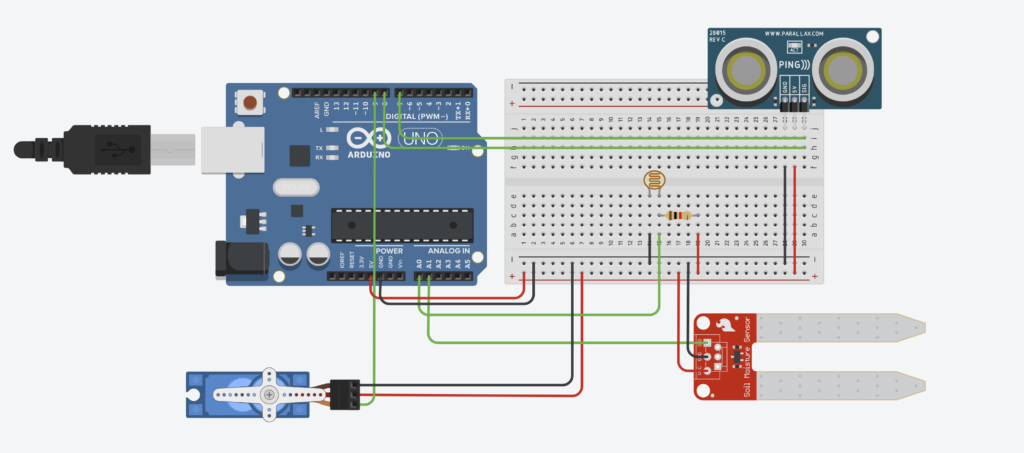
Note: Pin 7 and 8 are both attached to SIG in this diagram. In reality, “EchoPin” would be attached to Pin 7 and “TrigPin” attached to Pin 8. Additionally, the soil humidity sensor shown would be replaced by a water sensor.
Core Code
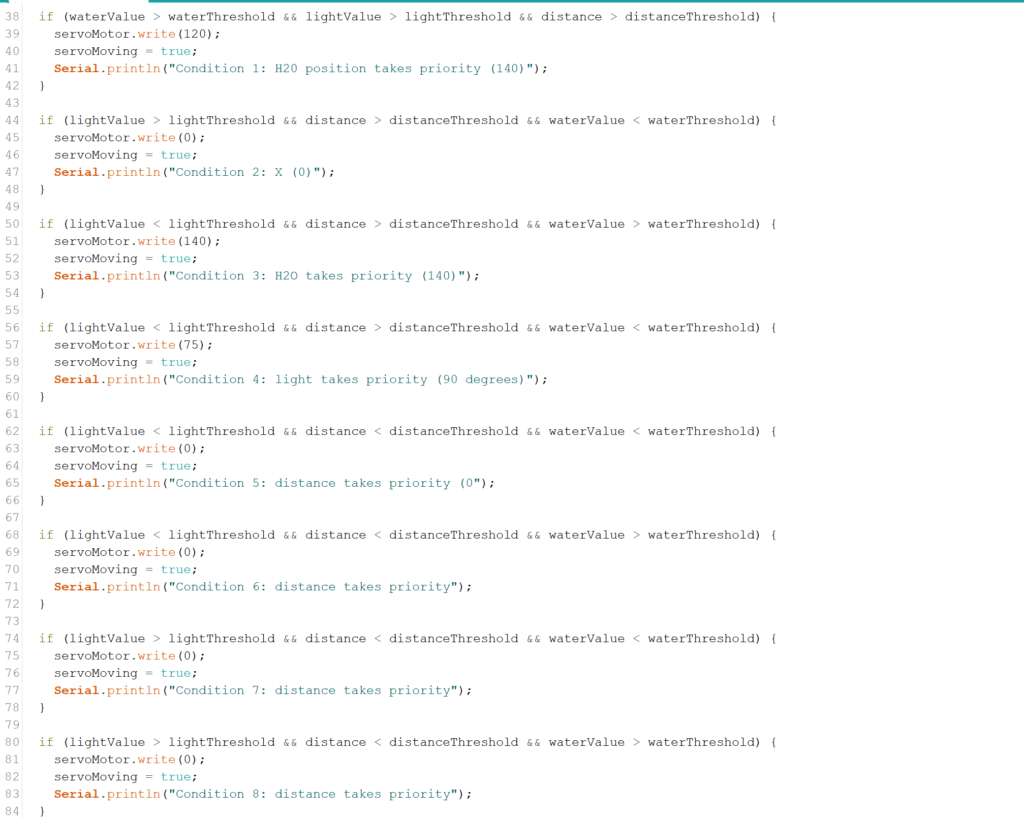
This section of code represents the fundamental behaviors of the system. Using a series of 8 “if” statements, the program was created to make the ultrasonic a kill switch to all other actions as a means of “protecting” the resources that the device would store. Furthermore, the position for rain collection takes priority over the solar positioning given the value of water in arid desert environments.
Prototype
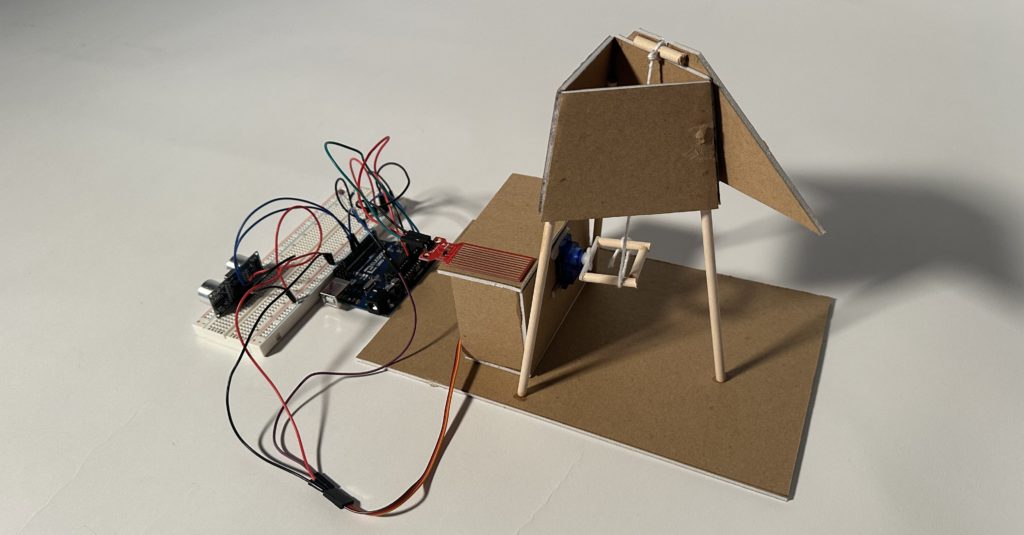
The prototype was developed to show the panel movement based on environmental factors and the. While the fully developed product would use more sophisticated motion elements, the servo motor adequately moves the position of the panel into the the three modes: protection (seen below), solar collection, and rain collection.
Solar Collection & Proximity Breach
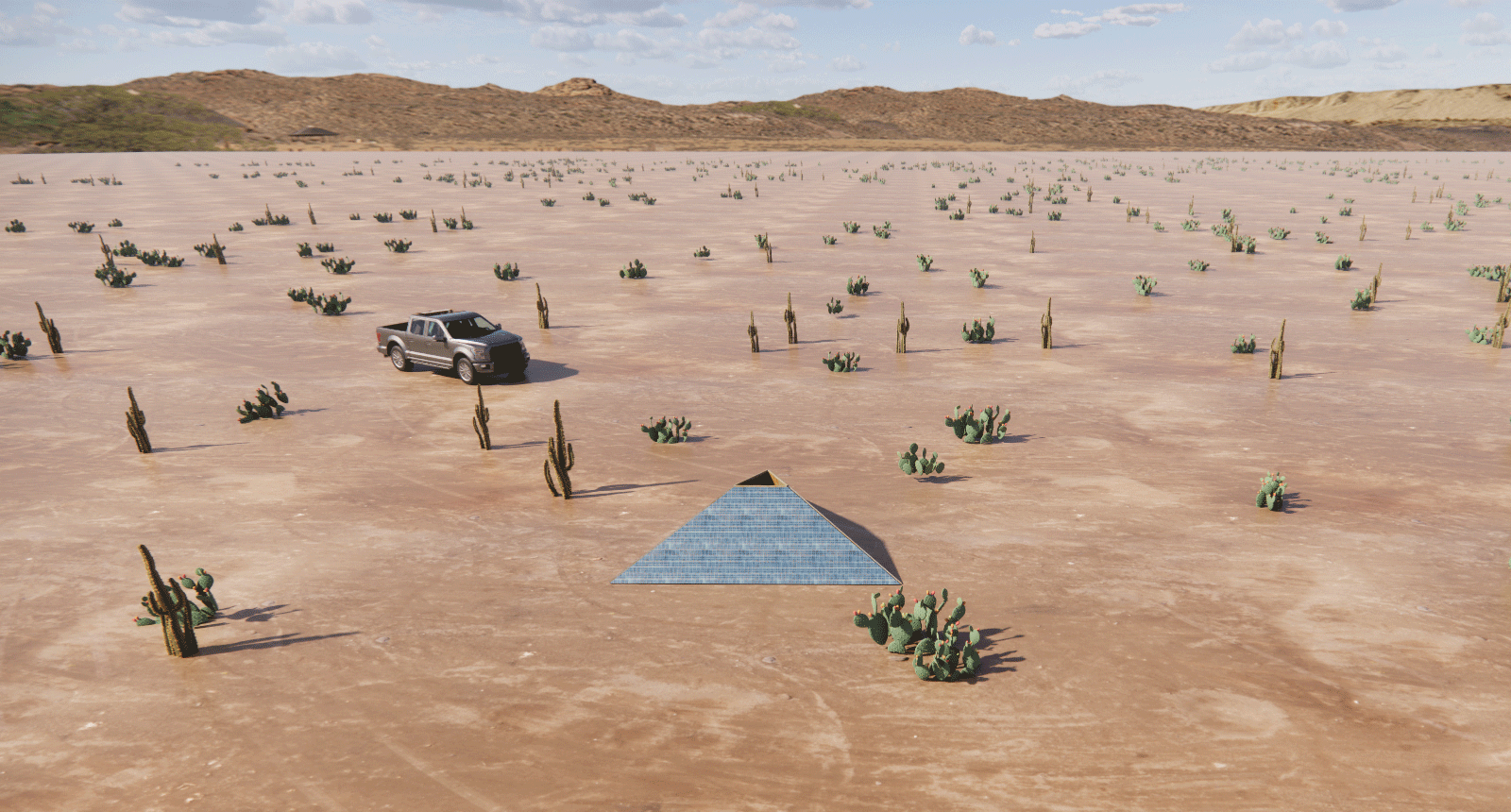
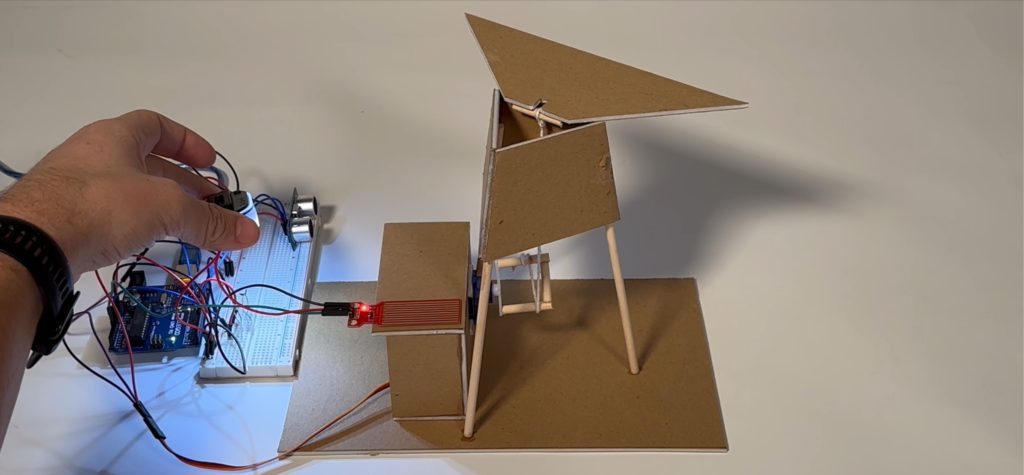
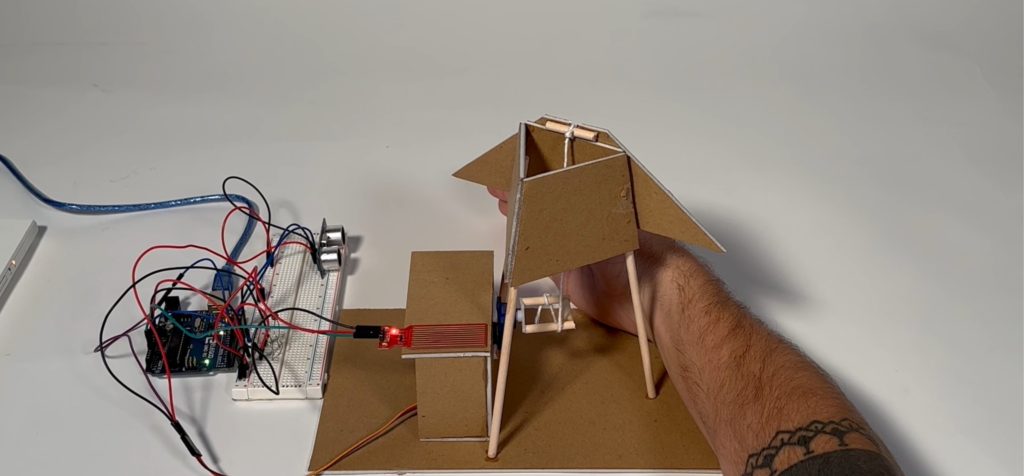
Rain Collection & Proximity Breach
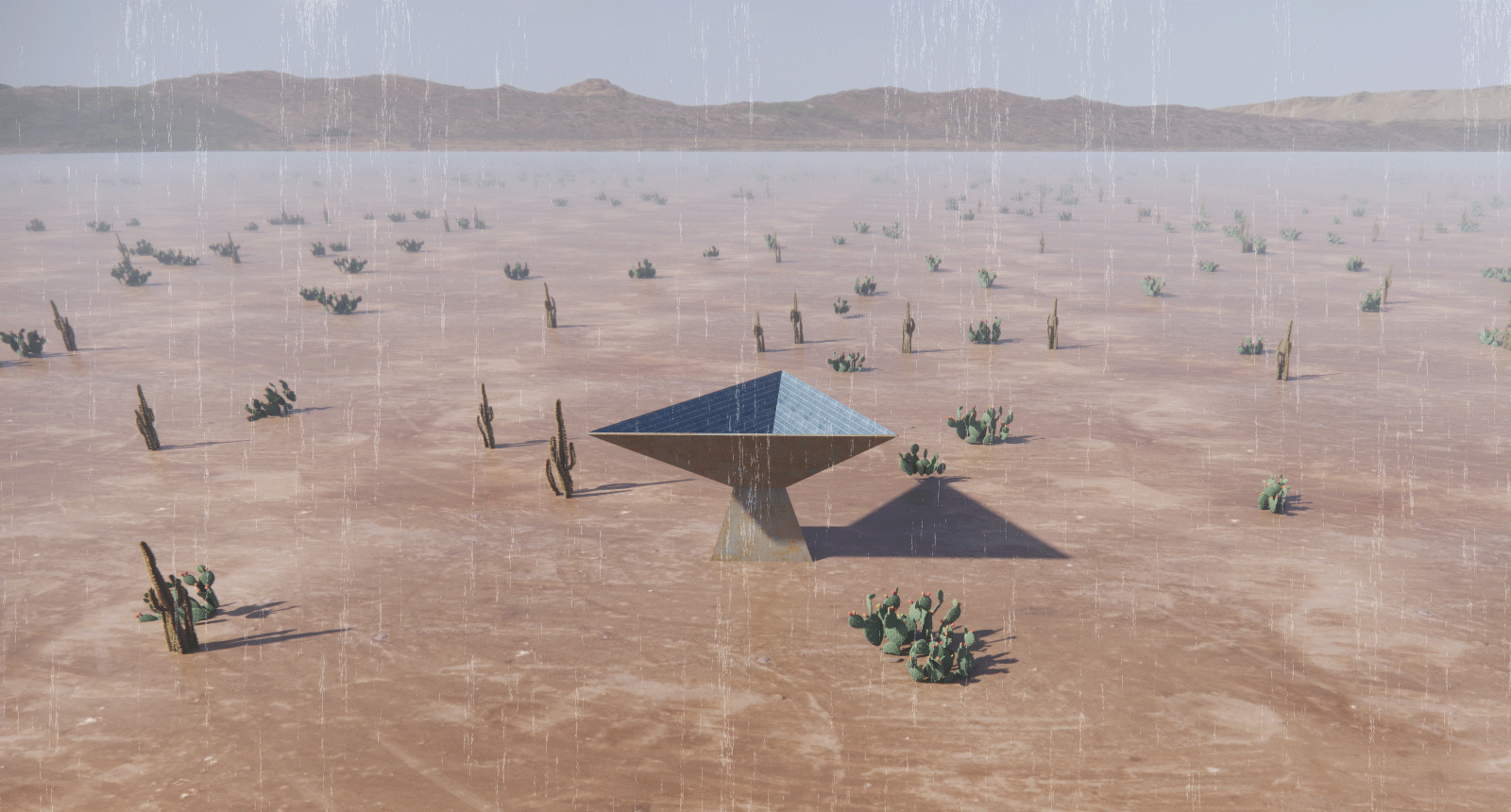
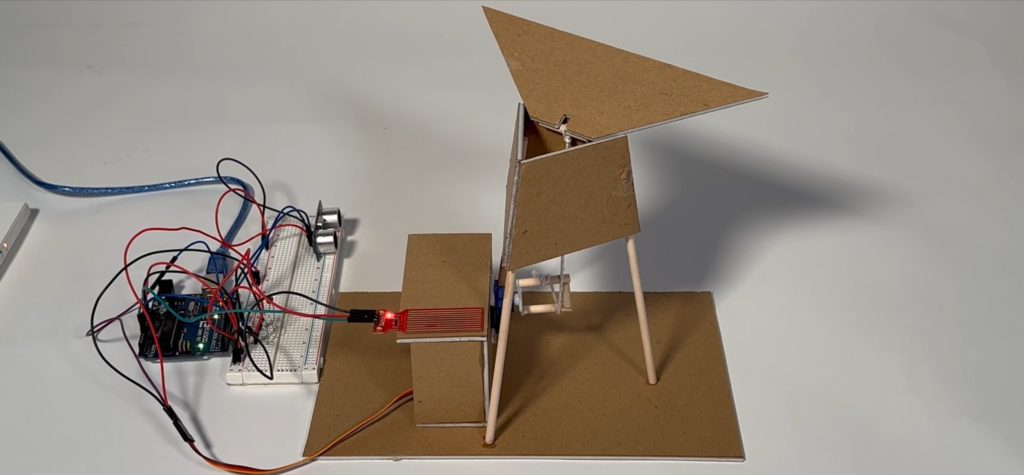
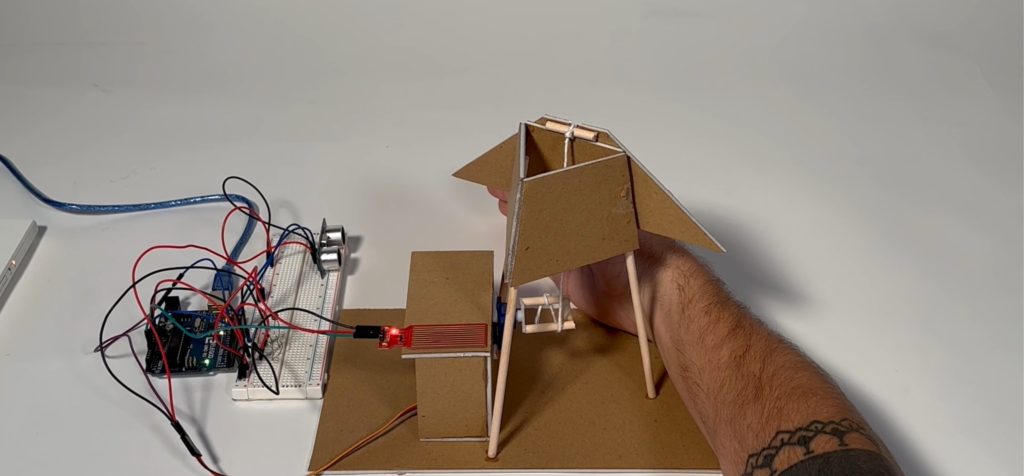
Presentation Video
Full Code
const int lightSensorPin = A0;
const int waterSensorPin = A1;
const int ultrasonicEchoPin = 7;
const int ultrasonicTrigPin = 8;
const int servoMotorPin = 9;
const int waterThreshold = 50;
const int lightThreshold = 100;
const int distanceThreshold = 15;
Servo servoMotor;
bool servoMoving = false;
void setup() {
servoMotor.attach(servoMotorPin);
pinMode(ultrasonicTrigPin, OUTPUT);
pinMode(ultrasonicEchoPin, INPUT);
Serial.begin(9600);
}
void loop() {
int lightValue = analogRead(lightSensorPin);
int waterValue = analogRead(waterSensorPin);
int distance = getUltrasonicDistance();
Serial.print(“Light Value: “);
Serial.print(lightValue);
Serial.print(” — Water Value: “);
Serial.print(waterValue);
Serial.print(” — Distance: “);
Serial.print(distance);
Serial.println(” cm”);
if (waterValue > waterThreshold && lightValue > lightThreshold && distance > distanceThreshold) {
servoMotor.write(120);
servoMoving = true;
Serial.println(“Condition 1: H20 position takes priority (140)”);
}
if (lightValue > lightThreshold && distance > distanceThreshold && waterValue < waterThreshold) {
servoMotor.write(0);
servoMoving = true;
Serial.println(“Condition 2: X (0)”);
}
if (lightValue < lightThreshold && distance > distanceThreshold && waterValue > waterThreshold) {
servoMotor.write(140);
servoMoving = true;
Serial.println(“Condition 3: H2O takes priority (140)”);
}
if (lightValue < lightThreshold && distance > distanceThreshold && waterValue < waterThreshold) {
servoMotor.write(75);
servoMoving = true;
Serial.println(“Condition 4: light takes priority (90 degrees)”);
}
if (lightValue < lightThreshold && distance < distanceThreshold && waterValue < waterThreshold) {
servoMotor.write(0);
servoMoving = true;
Serial.println(“Condition 5: distance takes priority (0”);
}
if (lightValue < lightThreshold && distance < distanceThreshold && waterValue > waterThreshold) {
servoMotor.write(0);
servoMoving = true;
Serial.println(“Condition 6: distance takes priority”);
}
if (lightValue > lightThreshold && distance < distanceThreshold && waterValue < waterThreshold) {
servoMotor.write(0);
servoMoving = true;
Serial.println(“Condition 7: distance takes priority”);
}
if (lightValue > lightThreshold && distance < distanceThreshold && waterValue > waterThreshold) {
servoMotor.write(0);
servoMoving = true;
Serial.println(“Condition 8: distance takes priority”);
}
delay(3000);
}
int getUltrasonicDistance() {
digitalWrite(ultrasonicTrigPin, LOW);
delay(2);
digitalWrite(ultrasonicTrigPin, HIGH);
delay(10);
digitalWrite(ultrasonicTrigPin, LOW);
long duration = pulseIn(ultrasonicEchoPin, HIGH);
int distance = duration / 60;
return distance;
}