The idea is to used the design module which created in G2- Bio tech morphogenesis studio and make a adaptive shading structure using Programming & Physical Computing.
Concept
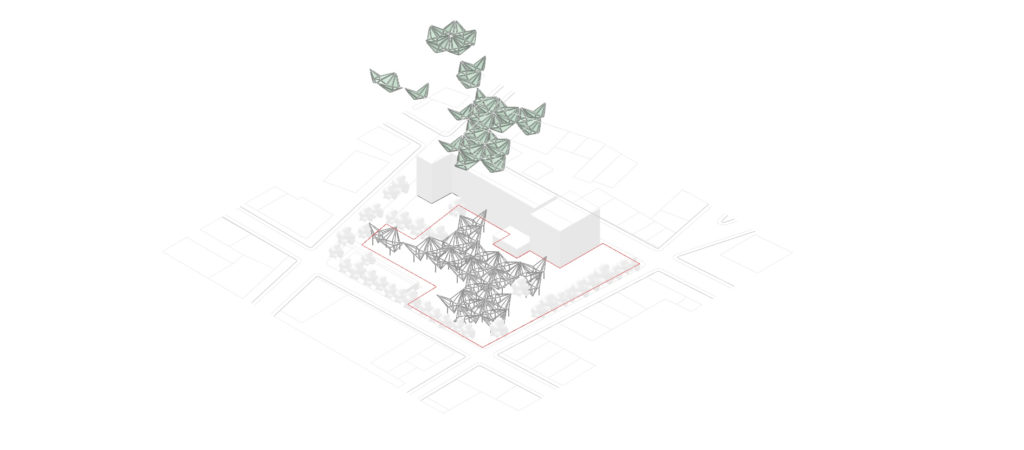
The Innovative algae panels integrated into structures enrich the surrounding air with oxygen through photosynthesis. Complementing this, kinetic shading devices dynamically adjust based on sunlight levels, efficiently curbing the urban heat island effect. This combined system not only enhances oxygen production but also optimizes shading for a sustainable and comfortable urban environment, showcasing a holistic approach to environmental design.
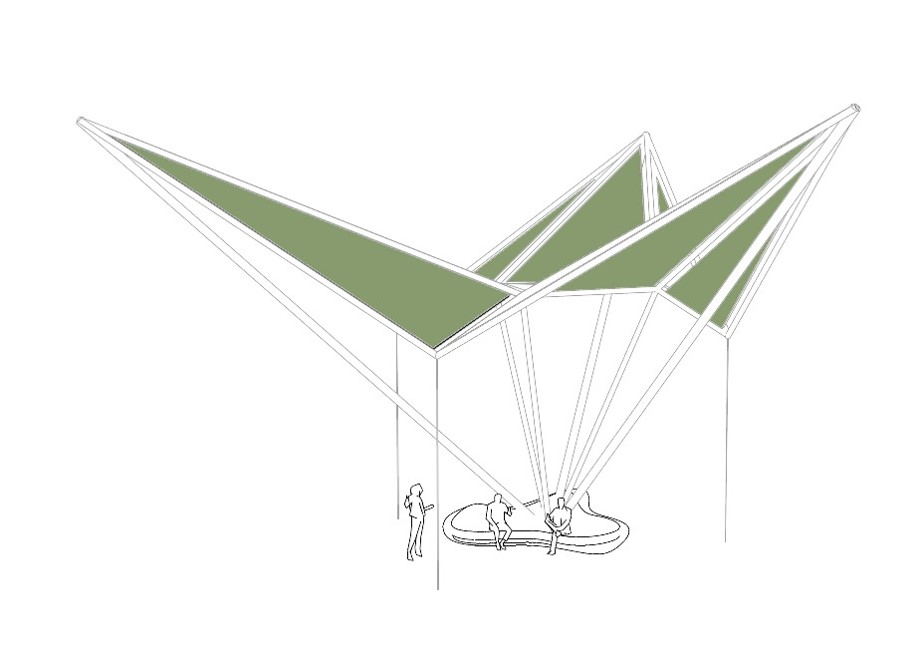
Process
Temperature:- Temperature measurements are taken using a DHT11 sensor, based on the temp the shading panel will open and close.
Air Quality:- Use of an Air Quality sensor to calculate the air quality and this helps to show the amount of air pure by the algae panels.
Sun Light:- Use of an LDR sensor to detect the amount of sunlight based on the amount of sun rays shading the device open and close. I.e.;- in the evening and in the morning shade is open but in afternoon its automatically close based on intensity of light.
Movement:- Use a Stepper motor to rotate the panels to close the shading device. It depends on the DHT11 and LDR sensor.
Required Arduino components
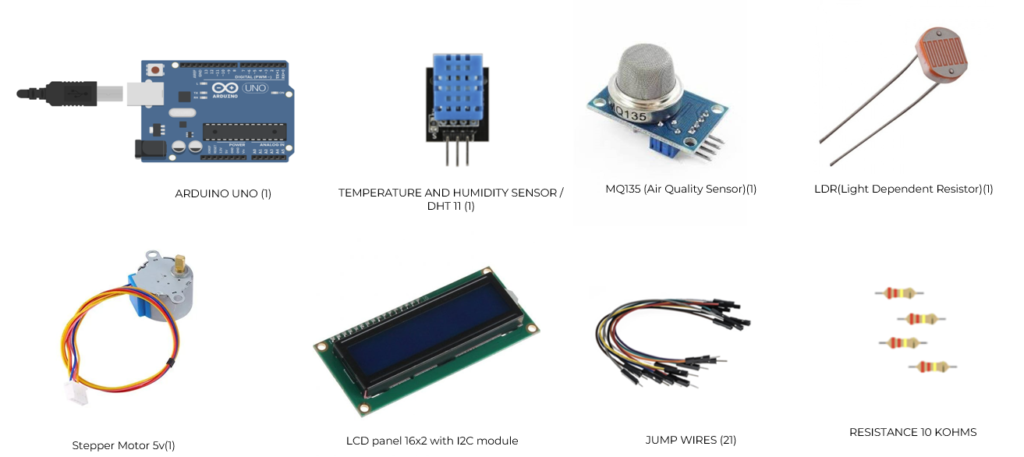
Circuit Diagram
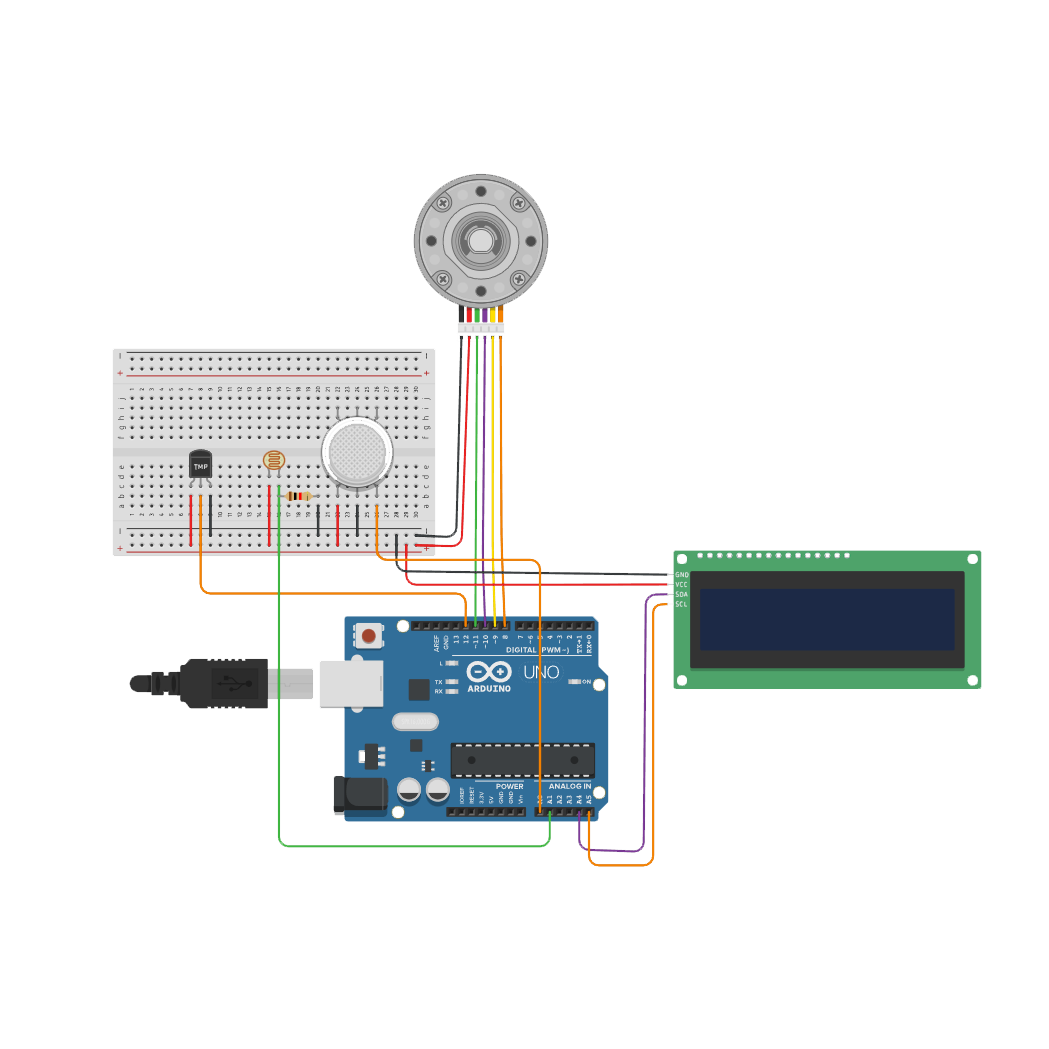
Input Data
1. DHT Sensor (Temperature and Humidity):
•Connected to pin 12 (DHTPIN).
•Sensor type is DHT11 (DHTTYPE).
2.Analog Air Quality Sensor:
•Connected to analog pin A0 (AirSensor).
3.Analog Light Dependent Resistor (LDR):
•Connected to analog pin A1 (LDRSensor).
4.Stepper Motor:
•Connected to pins 8, 9, 10, and 11 (IN1, IN2, IN3, IN4).
•Configured with 2048 steps per revolution (stepsPerRevolution).
5.LCD Display (I2C): Connected to I2C address 0x27.
C++ Arduino Code
#include “DHT.h”
#include<stepper.h>
#include<wire.h>
#include<LiquidCrystal_12C.h>
int AirSensor = A0;
int AirValue = 0;
int LDRSensor = A1; // Pin where the LDR is connected
int LDRValue = 0;
int IN1 = 8;
int IN2 = 9;
int IN3 = 10;
int IN4 = 11;
const int stepsPerRevolution = 2048;
int stepCount = 0;
DHT dht(DHTPIN, DHTTYPE);
Stepper stepper(stepsPerRevolution, IN1, IN3, IN2, IN4);
LiquidCrystal_I2C lcd(0x27,20,4);
void setup() {
Serial.begin(9600);
dht.begin();
stepper.setSpeed(15); // Set speed (RPM)
lcd.init();
lcd.backlight();
}
void loop() {
float temperature = dht.readTemperature();
delay(500);
Serial.println(“Entering loop”);
Serial.print(“Temperature: “);
Serial.print(temperature);
Serial.print(“\t “);
AirValue = analogRead(AirSensor);
Serial.print(“Air Quality: “);
Serial.print(AirValue);
Serial.print(“\t “);
LDRValue = analogRead(LDRSensor);
Serial.print(“Light Intensity: “);
Serial.println(LDRValue);
lcd.setCursor(2,0);
lcd.print(“Temp: “);
lcd.print(temperature);
lcd.setCursor(0,1);
lcd.print(“Air Quality: “);
lcd.print(AirValue);
if (temprature<21 && LDRValue < 900) {
Serial.println(“Rotating clockwise condition met”);
rotateStepperClockwise();
} else {
Serial.println(“Rotating anticlockwise condition met”);
rotateStepperAntiClockwise();
}
}
void rotateStepperClockwise() {
if (stepCount < 1) {
for (int i = 0; i < 3; ++i) {
stepper.step(stepsPerRevolution); // Rotate clockwise 360 degrees
delay(25);
}
stepCount++;
}
}
void rotateStepperAntiClockwise() {
if (stepCount > 0) {
for (int i = 0; i < 3; ++i) {
stepper.step(-stepsPerRevolution); // Rotate anti-clockwise 360 degrees
delay(25);
}
stepCount–;
}
}
Output Data
1. Serial Monitor:
A .Displays temperature, air quality, and light intensity values.
B. Prints rotation direction based on temperature and light intensity conditions.
2.LCD Display:
A. Shows temperature and air quality information.
3.Stepper Motor:
A .Rotates clockwise if temperature is below 21 degrees Celsius and light intensity is below 900.
B. Rotates anticlockwise otherwise.
Prototype Images
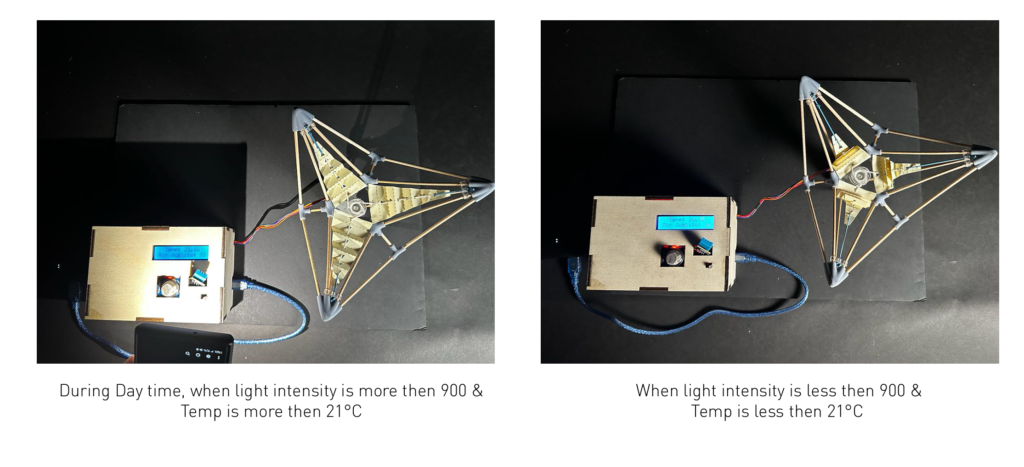
Prototype Video